Functional Interface :
A functional interface is an interface that contains exactly one abstract method. These interfaces can be instantiated using lambda expressions, method references, or anonymous classes. Functional interfaces are a key part of Java’s support for functional programming, especially since Java 8 introduced the Stream API and lambda expressions.@FunctionalInterface
interface MyFunctionalInterface {
void doSomething(String input);
interface MyFunctionalInterface {
void doSomething(String input);
}
Java Streams :
There are 2 types of java streams
Parallel Streams
Sequential Streams
Parallel Streams :
Java Streams provide a powerful way to process sequences of elements, and parallel streams allow you to perform operations on those elements concurrently, taking advantage of multiple CPU cores
A parallel stream divides the source data into multiple parts and processes each part in parallel using multiple threads. This can improve performance for large datasets, especially for CPU-intensive operations.
Method used in parallel streams
forEach,map,filter,collect,reduce,flatMap
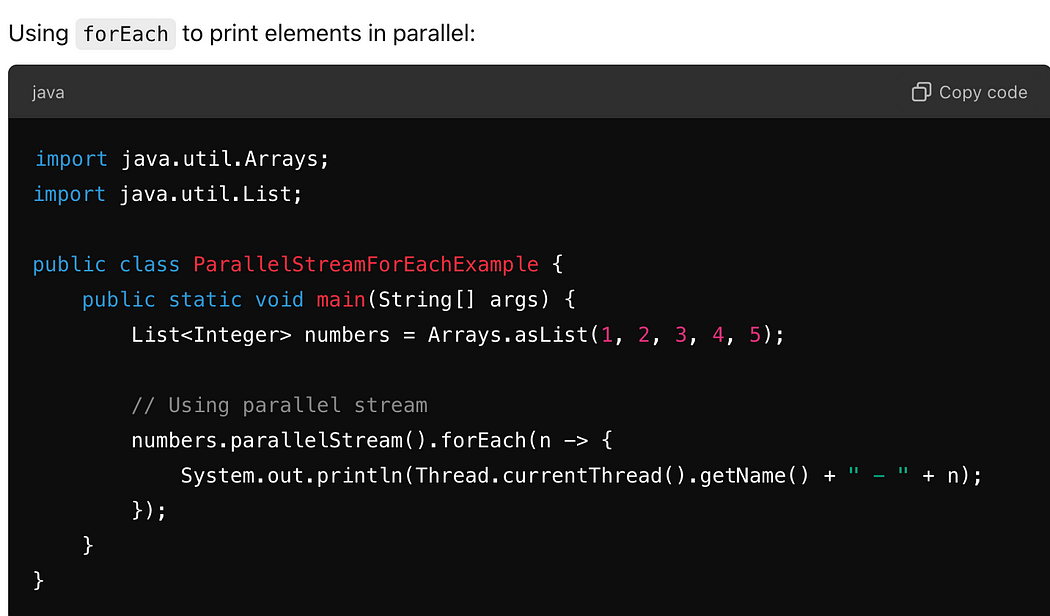
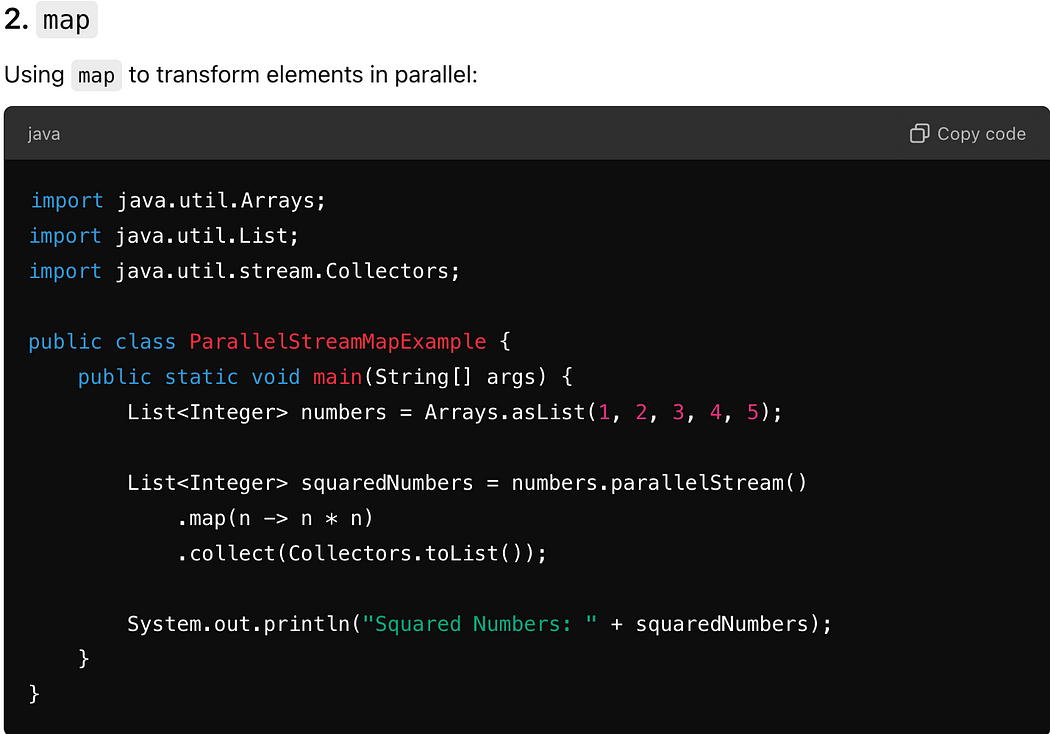

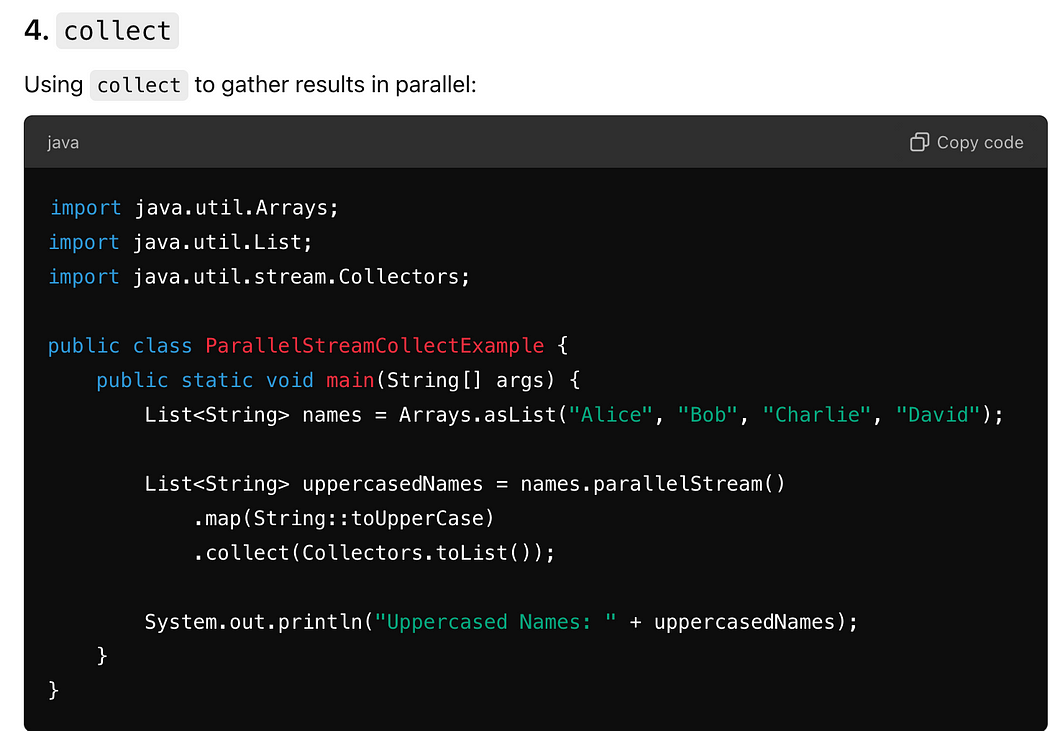
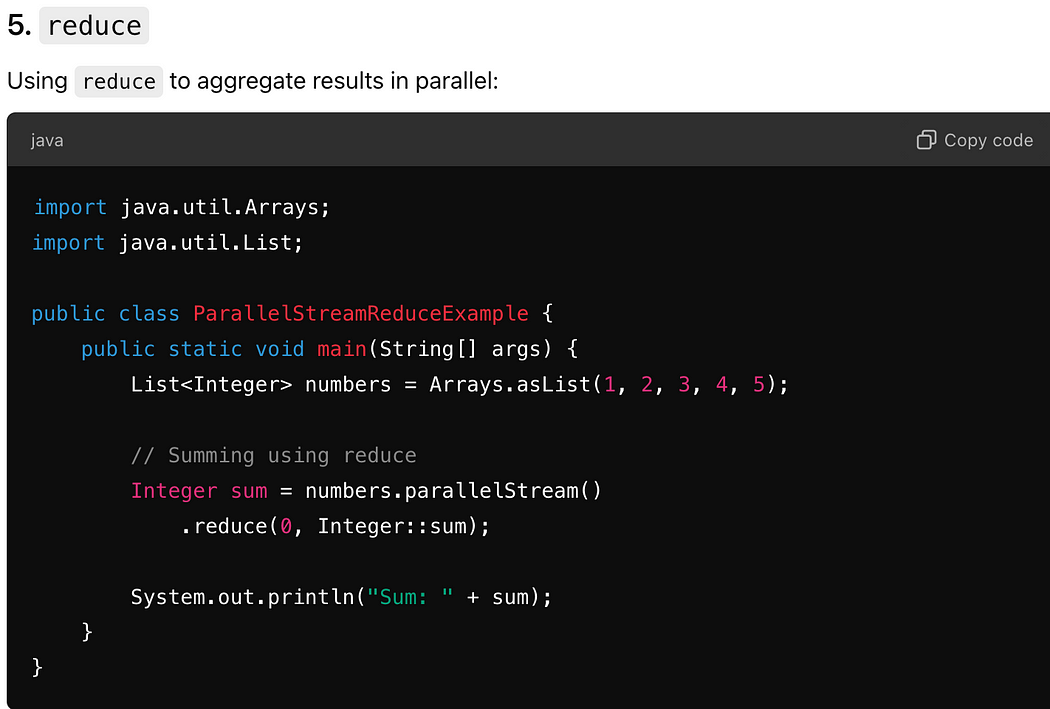
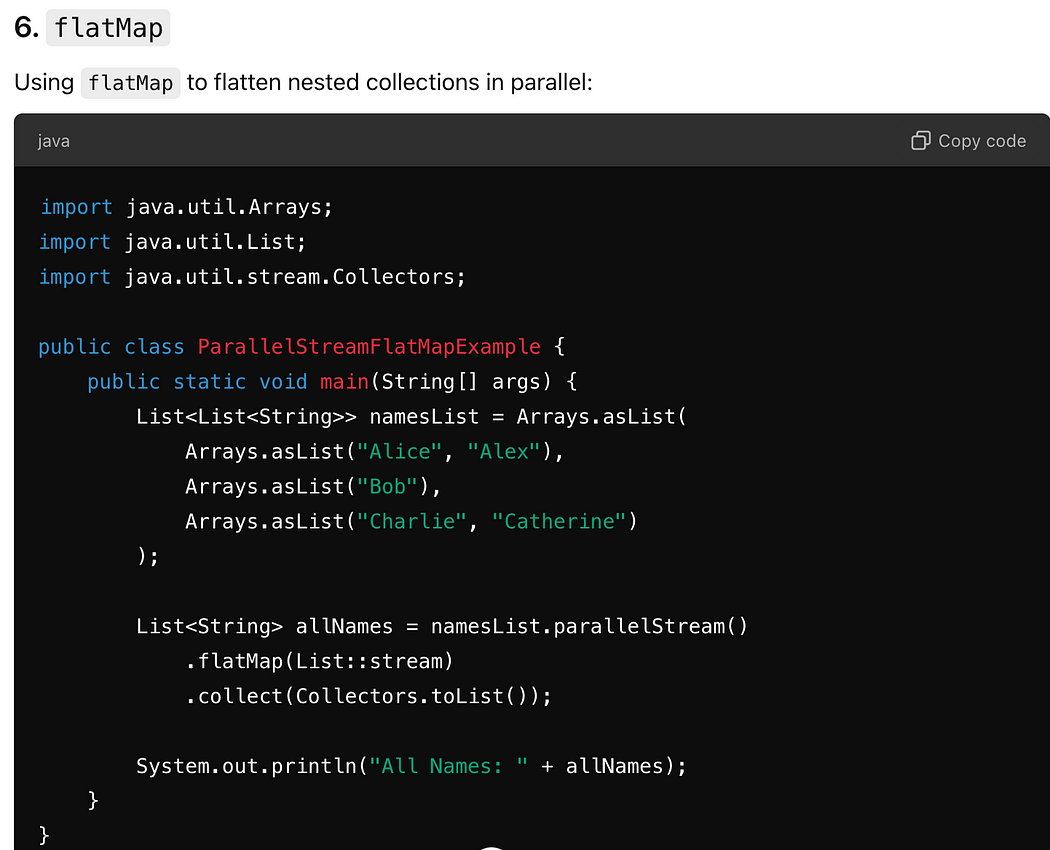
Sequential stream :
In Java, a sequential stream is the default type of stream processing, where elements are processed one at a time in the order they appear in the source. Sequential streams are simple to use and are suitable for many scenarios, especially when the dataset is small or when operations are not CPU-intensive.
Characteristics of Sequential Streams :
1. Single Threaded
2. Order of processing is maintained
3. Simple to understand
Operations on Sequential Streams :
Filtering: Using the filter method to select elements based on a condition.
Mapping: Using the map method to transform elements.
Collecting: Using the collect method to gather results into a collection.
Reducing: Using the reduce method to aggregate results.

There are 2 type of operations on streams
1. Terminal Operations
2. Intermediate Operations
Streams are kind of pipelines , which does not give any result if only intermediate operations are applied .
Streams will give result only when terminal operations are applied .
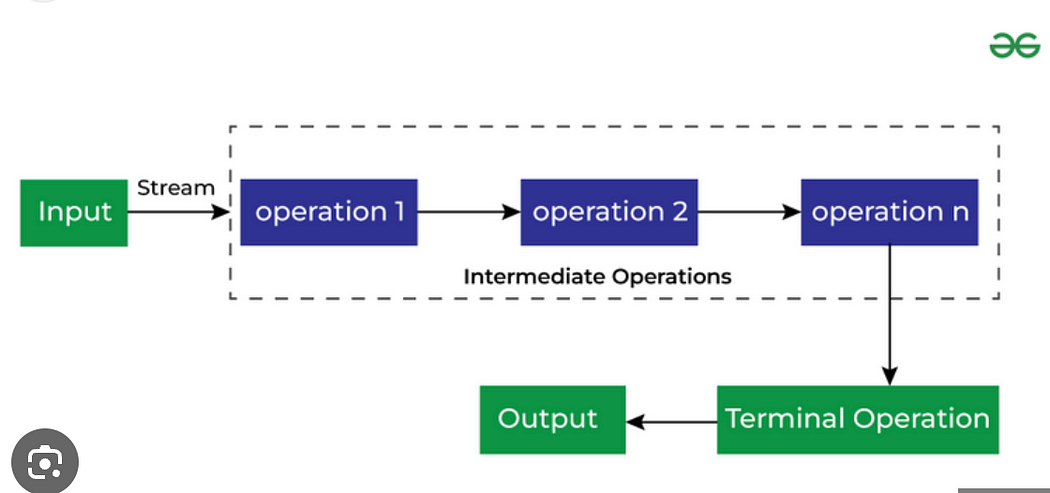

Example :
Intermediate Operations: filter(), map(), flatMap(), distinct(), sorted(), peek(), limit(), skip();
Terminal Operations: forEach(), collect(), reduce(), count(), min(), max(), anyMatch(), allMatch(), noneMatch(), findFirst(), findAny().
The most important questions is to tell the paramter type for each of the streams method .
Streams can be applied on :
Collections: Such as List, Set, Map, etc.
Arrays: Arrays of any type (e.g., int[], String[]).
I/O Channels: Such as files, using classes like Files.lines().
Generators: Using Stream.generate() or Stream.iterate().
Optional: The Optional class provides a stream of its value if present.
![]() |
Java Streams Operation Cheat-sheet |
Post a Comment